How do I resolve "Invalid permissions on Lambda function" errors from API Gateway REST APIs?
When I invoke my AWS Lambda function using an Amazon API Gateway REST API, I get an "Invalid permissions on Lambda function" error. What's causing the error, and how do I resolve it?
Short description
If your API Gateway REST API tries to invoke your Lambda function without Lambda invoke permission, then API Gateway returns the following error: "Invalid permissions on Lambda function"
If you set up CloudWatch logging for your REST API, then API Gateway also logs one of the following error messages:
CloudWatch error message example for REST APIs with a Lambda integration
"Sending request to https://lambda.xx.amazonaws.com/2015-03-31/functions/arn:aws:lambda:xx:xx:function:xxx/invocations
Execution failed due to configuration error: Invalid permissions on Lambda function
Method completed with status: 500"
CloudWatch error message example for REST APIs with a Lambda authorizer
"Sending request to https://lambda.xx.amazonaws.com/2015-03-31/functions/arn:aws:lambda:xx:xxx:function:xx/invocations
Execution failed due to configuration error: Invalid permissions on Lambda function
Execution failed due to configuration error: Authorizer error"
Resolution
To resolve these errors, do one of the following:
Add a resource-based Lambda invoke permission to your REST API using one of the methods described in this article.
-or-
Configure an AWS Identity and Access Management (IAM) execution role that grants your REST API permission to invoke your function.
For more information, see API Gateway permissions model for invoking an API.
Note:
- If you receive a 401 Unauthorized error, follow the instructions in Why am I getting API Gateway 401 Unauthorized errors after creating a Lambda authorizer?
- If you receive errors when running AWS Command Line Interface (AWS CLI) commands, make sure that you're using the most recent AWS CLI version.
To add Lambda invoke permission to a REST API with a Lambda integration using the API Gateway console
1. In the API Gateway console, on the APIs pane, choose the name of your REST API.
2. On the Resources pane, choose the configured HTTP method.
3. On the Method Execution pane, choose Integration Request.
4. For Integration type, choose Lambda Function.
5. Expand the Lambda Region dropdown list. Then, choose the AWS Region that your Lambda function is in.
6. Choose the Lambda Function dropdown list. Then, choose the name of your Lambda function.
7. A prompt appears that gives you the option to Add Permission to Lambda Function. Choose OK.
8. Choose Save. Then, choose Deploy the API to add the Lambda invoke permission to your API.
To add Lambda invoke permission to a REST API with a Lambda integration using a CloudFormation template
Add the following code snippet to your CloudFormation template:
Important: Replace <api-id> with your apiID. Replace the FunctionName value with the name of your Lambda function. Replace the SourceArn value with your API's source Amazon Resource Name (ARN).
SampleApiPermission: Type: AWS::Lambda::Permission Properties: Action: "lambda:InvokeFunction" FunctionName: !Ref MyLambdaFnA1 Principal: "apigateway.amazonaws.com" SourceArn: !Sub "arn:aws:execute-api:${AWS::Region}:${AWS::AccountId}:<api-id>/*/<method>/<resource>"
For more information on how to declare various CloudFormation template parts, see Template snippets.
To add Lambda invoke permission to a REST API with a Lambda integration using the AWS CLI
Run the following add-permission AWS CLI command:
Important: Replace the function-name value with the name of your Lambda function. Replace the source-arn value with the source ARN of your API. Replace the statement-id value with a statement identifier that differentiates the statement from others in the same policy.
aws lambda add-permission \ --function-name "$FUNCTION_NAME" \ --source-arn "arn:aws:execute-api:$API_GW_REGION:$YOUR_ACCOUNT:$API_GW_ID/*/$METHOD/$RESOURCE" \ --principal apigateway.amazonaws.com \ --statement-id $STATEMENT_ID \ --action lambda:InvokeFunction
To add Lambda invoke permission to a REST API with a Lambda authorizer using the API Gateway console
1. Create an IAM role for API Gateway that allows the "lambda:InvokeFunction" action. Then, copy the IAM role ARN to your clipboard.
2. In the API Gateway console, on the APIs pane, choose the name of your REST API.
3. On the Authorizers pane, choose the configured Lambda authorizer. Then, choose Edit.
4. For Lambda Invoke Role, enter the IAM role ARN you copied to your clipboard.
5. Choose Save. Then, choose Deploy the API.
To add Lambda invoke permission to a REST API with a Lambda authorizer using a CloudFormation template
Add the following code snippet to your CloudFormation template:
Important: Replace <api-id> with your apiID. Replace the FunctionName value with the name of your Lambda function. Replace <auth-id> with your Lambda authorizer's authorizerId.
SampleApiAuthPermission: Type: AWS::Lambda::Permission Properties: Action: "lambda:InvokeFunction" FunctionName: !Ref MyLambdaFnA1 Principal: "apigateway.amazonaws.com" SourceArn: !Sub "arn:aws:execute-api:${AWS::Region}:${AWS::AccountId}:<api-id>/authorizers/<auth-id>"
For more information on how to declare various CloudFormation template parts, see Template snippets.
To add Lambda invoke permission to a REST API with a Lambda authorizer using the AWS CLI
Run the following add-permission AWS CLI Command:
Important: Replace the function-name value with the name of your Lambda function. Replace the source-arn value with the source ARN of your API. Replace the statement-id value with a statement identifier that differentiates the statement from others in the same policy.
aws lambda add-permission \ --function-name "$FUNCTION_NAME" \ --source-arn "arn:aws:execute-api:$API_GW_REGION:$YOUR_ACCOUNT:$API_GW_ID/authorizers/$AUTHORIZER_ID" \ --principal apigateway.amazonaws.com \ --statement-id $STATEMENT_ID \ --action lambda:InvokeFunction
Related information
Related videos
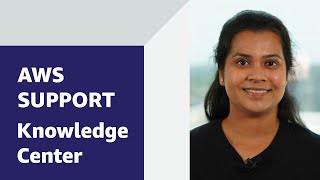

Relevant content
- asked a month agolg...
- asked 2 months agolg...
- asked 2 years agolg...
- AWS OFFICIALUpdated a year ago
- AWS OFFICIALUpdated 2 years ago
- AWS OFFICIALUpdated 2 years ago
- AWS OFFICIALUpdated a year ago